Selenium or Selenide: Which Testing Framework Best Fits Your Needs?
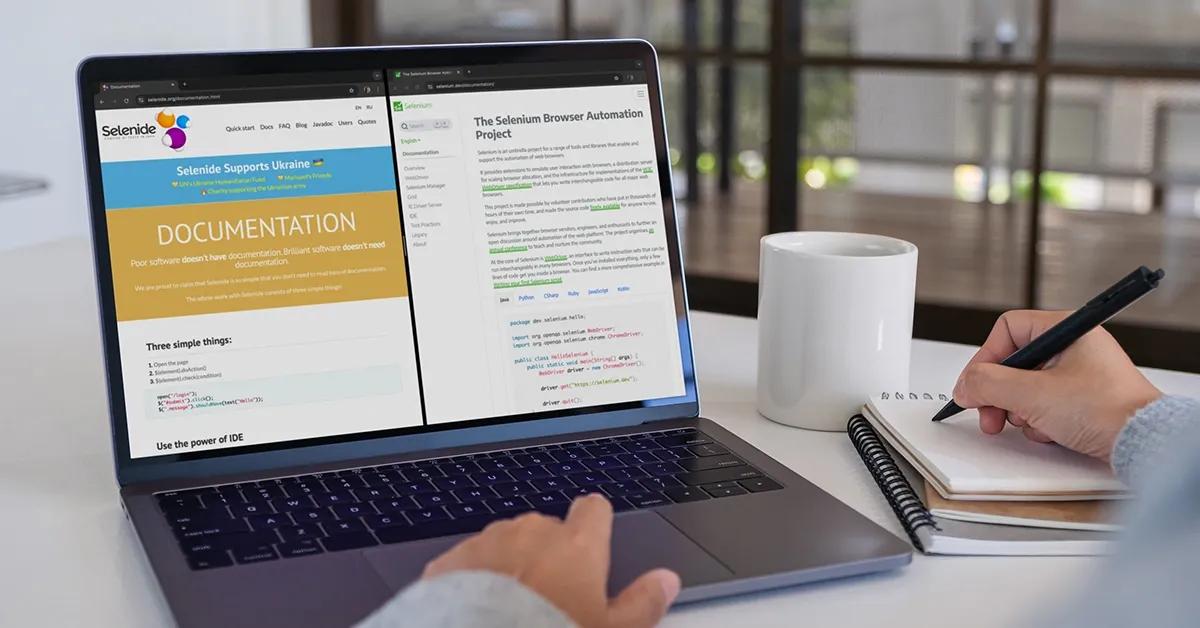
Automated testing is an essential practice in modern software development that ensures web applications function correctly across different environments and under various conditions. Selenium and Selenide are two popular test automation frameworks for web testing, each with their own unique features and benefits. Understanding their differences will help you make an informed decision about which tool to use.
In this article, we will discuss Selenium and Selenide, compare their key features, and analyze their pros and cons. We will also provide code examples and detailed comparisons to help you determine which framework best fits your needs.
What is Selenium?
Selenium is an open-source framework designed for automating web browsers. It is widely used for testing web applications, allowing developers and testers to create scripts that simulate user interactions with a web page. Selenium supports a wide range of programming languages, including Java, Python, C#, Ruby, and more. Selenium WebDriver, the core component of Selenium, allows for robust browser-based regression testing, enabling testers to simulate user interactions with web elements and validate the behavior of web applications.
Key features of Selenium:
- Cross-browser support. Works seamlessly with Chrome, Firefox, Safari, Edge, and others, ensuring compatibility across different environments.
- Multi-language support. Compatible with multiple programming languages, providing flexibility for diverse development teams.
- Flexibility and extensibility. Extensive API for fine-tuning and customizing test scripts to handle complex testing scenarios.
- Large community support. Access to extensive resources, plugins, and community support, making problem-solving easier and faster.
What is Selenide?
Selenide is a powerful wrapper around Selenium WebDriver that simplifies the process of writing automated tests. It provides a more concise and readable syntax, reducing boilerplate code and handling many common issues out-of-the-box, such as waiting for elements to appear or become clickable.
Key features of Selenide:
- Simplified syntax. More concise code with less boilerplate, making test scripts easier to write and maintain.
- Automatic waiting. Built-in waits for elements, reducing flakiness and improving test reliability.
- Readable code. Improved readability and maintainability, which is crucial for long-term projects.
- Java-based: Designed specifically for Java, leveraging its features and strengths for more powerful and efficient testing.
Selenium vs. Selenide
1. Ease of use
- Selenium: Requires more boilerplate code and a deeper understanding of its API. Testers need to handle waits and synchronization manually, which can increase complexity.
- Selenide: Offers a simpler, more readable syntax with built-in waits for elements. This reduces the amount of code and the potential for errors, making it easier for new testers to get started.
2. Functionality
- Selenium: Highly flexible and customizable. Developers can integrate various tools and libraries to extend its functionality, catering to complex and unique testing needs.
- Selenide: Focuses on ease of use and includes built-in solutions for common tasks like handling dynamic content and AJAX requests. This streamlines the testing process and reduces the need for additional code.
3. Performance
- Selenium: Performance can vary based on how well the tests are written, particularly in regards to handling waits and synchronization. Properly optimized tests can run efficiently, but this requires more effort.
- Selenide: Generally more reliable due to automatic waiting and simplified syntax, which reduces the likelihood of flaky tests and enhances overall test performance.
4. Integration
- Selenium: Widely used and integrates with a variety of tools and CI/CD pipelines, making it a versatile choice for diverse project requirements.
- Selenide: Also integrates well with CI/CD tools, but its simpler API can make integration faster and less error-prone, facilitating smoother DevOps workflows.
5. Community and support
- Selenium: Has a large and active community with extensive documentation and resources. This ensures that you can find help and solutions to problems quickly.
- Selenide: Has a smaller but growing community, with good documentation and focused support. The dedicated user base contributes to a friendly and supportive environment for troubleshooting.
6. Comparing Selenide and Selenium in practical scenarios
Asserting text in a list of web elements
Selenide: Selenide simplifies handling collections of elements. It provides concise ways to assert properties on all items in a collection.
$$(".item").shouldBe(CollectionCondition.textsInAnyOrder("Item1", "Item2", "Item3"));
Selenium:
With Selenium, you typically need to loop through elements and use a testing framework like JUnit or TestNG for assertions.
List<WebElement> items = driver.findElements(By.cssSelector(".item"));
List<String> expectedTexts = Arrays.asList("Item1", "Item2", "Item3");
List<String> actualTexts = items.stream().map(WebElement::getText).collect(Collectors.toList());
Assert.assertEquals(expectedTexts, actualTexts);
Asserting visibility after a series of actions
Selenide: Selenide handles conditions like visibility or invisibility straightforwardly after performing actions, automatically waiting for elements to satisfy conditions.
$("#button").click();
$("#modal").shouldBe(visible);
Selenium: In Selenium, you need to explicitly wait for elements to meet conditions after interactions.
driver.findElement(By.id("button")).click();
new WebDriverWait(driver, Duration.ofSeconds(10)).until(ExpectedConditions.visibilityOfElementLocated(By.id("modal")));
Checking for absence or disappearance
Selenide: Checking for an element's absence or disappearance is directly supported with concise syntax.
// Check that an error message eventually disappears
$("#error").should(disappear);
Selenium: This requires setting up explicit waits to assert that an element is no longer visible or present.
new WebDriverWait(driver, Duration.ofSeconds(10)).until(ExpectedConditions.invisibilityOfElementLocated(By.id("error")));
Asserting complex conditions with custom messages
Selenide: You can create complex conditions and attach custom failure messages for better debugging.
$("#submit").shouldBe(enabled.and(visible).because("The submit button should be enabled and visible after form completion"));
Selenium: With Selenium, custom messages are typically appended to assertions within the testing framework being used.
WebElement submit = driver.findElement(By.id("submit"));
boolean isEnabledAndVisible = submit.isEnabled() && submit.isDisplayed();
Assert.assertTrue(isEnabledAndVisible, "The submit button should be enabled and visible after form completion");
Chaining assertions for a sequence of states
Selenide: Chain conditions to assert a sequence of states on a single element, making it easy to follow.
// Verifying an input field is empty, filled, and then cleared
$("#input").should(be(empty))
.setValue("Hello World")
.shouldHave(value("Hello World"))
.clear();
$("#input").shouldBe(empty);
Selenium: Each state needs individual assertions, typically with temporary variables or repeated element location
WebElement input = driver.findElement(By.id("input"));
Assert.assertEquals(input.getAttribute("value"), "");
input.sendKeys("Hello World");
Assert.assertEquals(input.getAttribute("value"), "Hello World");
input.clear();
Assert.assertEquals(input.getAttribute("value"), "");
Pros and Cons

Advantages of Selenium
- Supports multiple programming languages, offering flexibility for diverse development environments.
- Extensive community and resources, providing ample support and solutions.
- Highly flexible and customizable, allowing for tailored testing solutions.
Disadvantages of Selenium
- Steeper learning curve, which can be challenging for beginners.
- Requires more boilerplate code, leading to potentially longer development times.
- Manual handling of waits and synchronization, increasing complexity and risk of flaky tests.
Advantages of Selenide
- Simpler and more readable syntax, making it easier to write and maintain tests.
- Built-in support for dynamic content and AJAX, reducing the need for additional code.
- Reduces boilerplate code and potential for errors, improving development efficiency.
Disadvantages of Selenide
- Limited to Java, which may not be suitable for teams using other programming languages.
- Smaller community compared to Selenium, which can affect the availability of resources and support.
Use cases
Use cases for Selenium and Selenide
Selenium is ideal for projects that require support for multiple programming languages, providing flexibility for diverse development teams. It is well suited for complex testing scenarios needing extensive customization, allowing for tailored testing solutions.
On the other hand, Selenide is perfect for projects that prioritize ease of use and maintainability, improving development efficiency. It benefits teams looking to reduce test maintenance effort and as a result save time and resources. Also, Selenide is particularly advantageous for applications with dynamic content and AJAX, thanks to its built-in support and automatic waiting features.
Key takeaways
Both Selenium and Selenide have their strengths and are suitable for different scenarios. Selenium offers greater flexibility and a larger community, making it ideal for complex projects requiring extensive customization. In contrast, Selenide simplifies the testing process, making it a great choice for projects where ease of use and maintainability are critical. Ultimately, the choice between Selenium and Selenide should be based on your specific project requirements and team expertise.
By understanding the unique advantages of each framework, you can make an informed decision and choose the tool that best fits your needs, ensuring the success and reliability of your web application testing efforts.
Do you want to implement test automation into your testing project? We can help you get started and ensure you choose the right framework, whether it's Selenium, Selenide, or another tool. Get in touch to learn more about our test automation services.