Integration Testing 101: Definition, Best Practices, and Examples
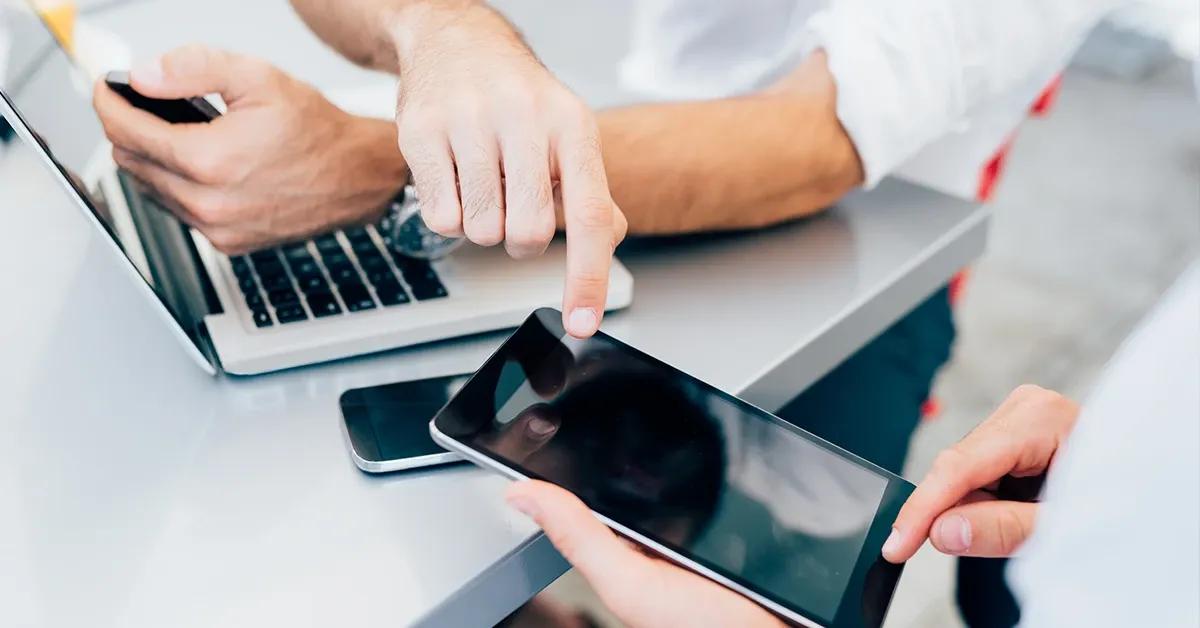
Integration testing is a type of software testing that helps detect interface issues early, ensures component compatibility, improves software quality, reduces the risk of system failures, and facilitates easier debugging. By integrating and testing modules as they are developed, teams can identify and resolve issues before they escalate, thereby enhancing the overall reliability and performance of the software. This comprehensive guide delves into the definition, best practices, and examples of integration testing, highlighting its importance in modern software development.
What is integration testing?
Integration testing is an approach that checks how different parts of a software application work together. The main goal of integration testing is to verify that the different modules or services within a system work together as expected. This type of testing is crucial because while individual units may function correctly on their own, problems can arise when they interact with each other. But more on the importance of integration testing below.
Why is integration testing important?
Integration testing is important in the software development process for several reasons. Here are the top five:
1. Detects interface issues early.
Integration testing helps identify issues at the interfaces of different modules. These issues might not be evident during unit testing when modules are tested in isolation. Early detection of such issues can prevent more significant problems later in the development cycle.
2. Ensures component compatibility.
Integration testing verifies that different modules or components work together as intended. This ensures that integrated components interact correctly, reducing the risk of integration problems when the system is deployed.
3. Improves software quality.
Integration testing contributes to higher software quality by catching defects early. It ensures that the system's modules work together seamlessly, which is critical for the software's overall functionality and performance.
4. Reduces risk of system failures.
Integration testing helps uncover critical issues that might cause system failures. By identifying and addressing these issues early, developers can reduce the risk of failures in production, leading to a more reliable and stable software product.
5. Facilitates easier debugging.
Issues identified during integration testing are easier to isolate and debug because the scope of the code being tested is smaller than in system testing. This focused approach can save time and effort when troubleshooting and fixing defects.
Challenges in integration testing

Integration testing comes with its own set of challenges. Here are some of the key challenges you might face during integration testing:
Complexity
As integration testing focuses on how various components interact, it can become complicated, especially for projects with many interconnected parts. This complexity can lead to time-consuming test creation and execution.
Dependency management
Integration tests often rely on all the software components being available. If components are missing or under development, testers might need to create stubs or mocks to simulate their behavior. Managing these dependencies can add extra effort to the testing process.
Environment setup
Integration testing often requires a specific testing environment that mimics the production environment as closely as possible. Setting up and maintaining this environment can be challenging, especially if it involves multiple systems or external dependencies.
You might be interested in: Introducing LiveLy Box: Our New Custom Test Environment for Video Quality Testing
Legacy system integration
Integrating new systems with legacy systems can be particularly tricky. Legacy systems might have different coding standards, data formats, or functionalities, requiring extra effort to ensure proper interaction during testing.
Test maintenance
As software evolves and new features are added, integration tests need to be updated accordingly. Maintaining a large suite of integration tests can be time-consuming and requires ongoing effort throughout the development lifecycle.
Common approaches to integration testing
There are several ways you can approach integration testing, each with its own advantages and disadvantages. Here are some of the most common ones:
Top-down approach
This approach starts with testing the high-level modules or components that rely on lower-level ones. Stubs (simulated lower-level modules) are used to isolate and test the top-level functionality. This approach is good for testing the overall system flow but can make it difficult to pinpoint the exact source of an issue.
Bottom-up approach
This approach starts by testing the low-level, independent modules and gradually integrates them to form larger components. Drivers (simulated higher-level modules) interact with and test the lower-level modules. This approach is good for ensuring individual component functionality but might not effectively test the overall system flow until later stages.
Incremental approach
This approach combines elements of both top-down and bottom-up approaches. Modules are integrated and tested in small increments, with both top-level and lower-level modules gradually tested together. This approach provides a balance between testing individual components and overall system flow.
Big bang approach
This is a simpler approach in which all components are integrated at once and then tested as a whole system. This can be fast for small projects, but it can be difficult to isolate and debug issues due to the complexity of interactions between all components.
Sandwich testing (hybrid approach)
This approach combines top-down and bottom-up testing strategies. Core functionalities are tested using a bottom-up approach, while the overall system flow is validated with top-down testing. This approach offers a balance between the two individual approaches.
Approach | Top-down integration testing | Bottom-up integration testing | Incremental integration testing | Big bang integration testing | Sandwich (hybrid) integration testing |
---|---|---|---|---|---|
Description | Starts with top-level modules and integrates downward | Starts with lower-level modules and integrates upward | Modules integrated and tested incrementally | All modules integrated simultaneously | Combines top-down and bottom-up approaches |
Fault isolation | Moderate, high-level issues found early | Good, low-level issues found early | Good, issues found in smaller sets of modules | Poor, difficult to pinpoint faults | Good, issues found at multiple levels |
Need for stubs/drivers | Requires many stubs for lower modules | Requires many drivers for higher modules | May need both stubs and drivers | No stubs or drivers are needed initially | Requires both stubs and drivers |
Early testing of | High-level design and architecture | Low-level functionalities | Specific, functional/logical groupings | The entire system is tested at once | Both high-level and low-level functionalities tested early |
Complexity | Moderate | Moderate | Low to moderate, depending on the strategy | Low initially, high overall | High, requires careful planning |
Resource requirements | High, due to stubs | High, due to drivers | Moderate, balanced approach | High, significant resources needed for comprehensive testing | High, due to the need for both stubs and drivers |
Risk of missing defects | Moderate | Low, at lower levels | Low, as modules are tested incrementally | High, due to simultaneous integration | Low to moderate, balanced detection |
Suitability | Systems with well-defined high-level modules | Systems with robust low-level modules | Systems with well-defined interfaces and dependencies | Small, less complex systems | Complex systems with multiple layers |
Example use cases | Enterprise applications with a clear hierarchy | Utility libraries, framework development | Web applications, service-oriented architectures | Small applications, prototyping | Complex distributed systems |
Each approach has its own strengths and weaknesses, and the choice of which to use can depend on the project's specific needs and context. In many cases, you can use a combination of these approaches to effectively cover different aspects of the system being tested.
What are the key steps in integration testing?
The key steps in integration testing are designed to ensure that the various components of a software system work together as intended. Here is a short step-by-step guide to the integration testing process:
Step 1: Plan the integration testing
- Determine which modules or components will be integrated and tested.
- Identify the interfaces between modules that need to be tested.
- Choose the appropriate integration testing approach (e.g., top-down, bottom-up, incremental, big bang, or sandwich).
Step 2: Design test cases
- Develop test scenarios based on the interactions between modules.
- Identify or create the test data needed to execute the test cases.
- Write automated test scripts or prepare manual test cases for integration tests.
Step 3: Set up the test environment
- Set up the hardware, software, network, and other configurations needed to replicate the production environment.
- Install and configure any testing tools required to execute the tests.
- Ensure that the test environment is stable and ready for testing.
Step 4: Execute integration tests
- Combine the modules or components based on the chosen integration strategy.
- Execute the test cases designed for integration testing.
- Monitor the execution and log the results of each test case, noting any issues or failures.
Step 5: Analyze test results
- Review the logs to identify any issues or defects that occurred during testing.
- Categorize and prioritize the defects based on their impact and severity.
- Record the outcomes of the tests, including any defects found, in a test report.
Step 6: Debugging and fixing defects
- Investigate the root causes of the defects identified during testing.
- Work with the development team to resolve the defects.
- Re-run the failed test cases to ensure that the issues have been fixed and that no new issues have been introduced.
Step 7: Opt for regression testing
- Execute a set of regression tests to ensure that the recent changes have not adversely affected existing functionality.
- Confirm that all defects have been fixed and that the system behaves as expected.
Step 8: Create reporting and documentation
- Compile a detailed test report summarizing the integration testing process, including test cases executed, defects found and fixed, and overall system stability.
- Document any lessons learned, best practices, and any updates needed for future integration testing efforts.
Following these steps helps ensure a thorough approach to integration testing, reduce the risk of integration-related issues, and improve the overall quality of the software system.
Best practices for integration testing
Here are some best practices for integration testing:
- Integrate and test modules as soon as they are developed to identify issues early.
- Prioritize testing the most crucial interactions between components that directly affect core functionalities.
- Isolate components under test by mocking or stubbing external dependencies (databases, APIs) to avoid reliance on external factors during testing.
- Automate repetitive integration tests using frameworks or tools to improve efficiency, reduce human error, and enable faster feedback loops.
- Structure integration tests to be modular and independent, focusing on specific interactions between components. This improves the maintainability and reusability of tests.
- Based on your project structure and dependencies, consider a strategic combination of top-down and bottom-up approaches.
- Write clear, concise, and well-documented test cases that outline the purpose, steps, expected results, and any assumptions made. This enhances clarity and facilitates collaboration.
- Re-run integration tests after code changes or bug fixes to ensure the changes haven't caused regressions or introduced new integration problems.
- Design test cases that verify how components handle errors, unexpected inputs, or edge cases (boundary conditions) to ensure system robustness.
- Foster close collaboration between development and testing teams to ensure effective communication, early problem identification, and a shared understanding of testing objectives.
- Ensure that all modules have been unit-tested before moving to the integration phase so that the integration test results are reliable.
- Validate the input test data for higher test reliability.
Top 5 tools for integration testing

Choosing the right integration testing tool depends on the project's specific requirements, including the type of application, the testing environment, and the integration needs. Let’s take a quick look at the top 5 most commonly used testing tools:
Jenkins
Jenkins is a leading open-source automation server that facilitates continuous integration and continuous delivery. It automates the parts of software development related to building, testing, and deploying, facilitating integration testing within CI/CD pipelines.
Selenium
Selenium is a suite of tools for automating web browsers. It is primarily used for automating web application testing but can also be extended for integration testing.
JUnit
JUnit is a widely-used testing framework for Java. While it is primarily intended for unit testing, it can be used in conjunction with other tools for integration testing.
Postman
Postman is a collaboration platform for API development. It simplifies the process of developing, testing, and documenting APIs, making it suitable for integration testing of APIs.
Apache JMeter
Apache JMeter is an open-source tool designed to load test functional behavior and measure performance. While it is mainly used for performance testing, it can also be used for integration testing by simulating complex scenarios.
Description | Jenkins | Selenium | JUnit | Postman | Apache JMeter |
---|---|---|---|---|---|
Purpose | Continuous Integration (CI) and Continuous Delivery (CD) | Web application testing automation | Unit testing framework for Java | API testing and development | Load testing and performance testing |
Primary use Case | Automating the build, integration, and deployment processes | Automating browser interactions for web applications | Writing and running unit tests for Java applications | Testing RESTful APIs and web services | Testing the performance and scalability of applications |
Integration testing | Facilitates integration testing by running automated tests | It can be used for integration testing of web-based applications | Used in combination with other tools for integration tests | Used for integration testing of APIs | It can be used for integration testing by simulating complex scenarios |
Complexity | Moderate to low | Moderate to low | Low | Low | Moderate to low |
Language support | Supports multiple languages via plugins | It supports multiple languages (Java, Python, C#, etc.). | Primarily Java | Supports multiple languages via scripting | Java, with plugins for other languages |
Automation | Extensive automation capabilities through plugins | Extensive support for test automation | Automation of unit tests, extendable for integration tests | Strong automation capabilities for API testing | Extensive automation for load and performance tests |
Continuous Integration | Excellent integration with CI/CD pipelines | It can be integrated with CI tools like Jenkins | It can be integrated with CI tools like Jenkins | It can be integrated with CI tools like Jenkins | It can be integrated with CI tools like Jenkins |
Test reporting | Detailed reports and dashboards | Detailed test reports support third-party reporting tools | Provides test results and reports | Detailed reports on API tests | Detailed performance test reports and analysis |
Community support | Large, active community and extensive plugin ecosystem | Large, active community with extensive documentation | Large community, especially within the Java ecosystem | Large, active community with extensive resources | Large community with extensive resources |
Cost | Open source | Open source | Open source | Open source | Open source |
Integration testing example
Here’s a practical example of an integration testing scenario for a web application that includes a front-end, a back-end API, and a database. We will use Jenkins, Selenium, and JUnit to demonstrate the integration testing process.
The scenario:
A web application for an online bookstore. The application consists of:
Front-end: a web interface where users can browse and purchase books.
Back-end API: a RESTful API that handles book information, user authentication, and order processing.
Database: a database that stores user data, book details, and order information.
Steps for integration testing:
Step 1: Set up Jenkins for continuous integration
Download and install Jenkins on a server and configure a Jenkins pipeline to automate the build, test, and deployment processes.
pipeline {
agent any
stages {
stage('Build') {
steps {
// Code to compile and build the project
sh 'mvn clean install'
}
}
stage('Integration Tests') {
steps {
// Run integration tests
sh 'mvn verify'
}
}
stage('Deploy') {
steps {
// Code to deploy the application
sh 'mvn deploy'
}
}
}
}
Step 2: Write integration test cases with JUnit
Write JUnit test cases to test the integration between different modules, for example, testing the user registration API and verifying data in the database.
import static org.junit.Assert.*;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
@SpringBootTest
public class UserIntegrationTest {
@Autowired
private RestTemplate restTemplate;
@Test
public void testUserRegistration() {
String url = "http://localhost:8080/api/register";
User user = new User("testuser", "password", "testuser@example.com");
ResponseEntity<User> response = restTemplate.postForEntity(url, user, User.class);
// Verify HTTP status code
assertEquals(HttpStatus.OK, response.getStatusCode());
// Verify user data in the database
User registeredUser = userRepository.findByUsername("testuser");
assertNotNull(registeredUser);
assertEquals("testuser@example.com", registeredUser.getEmail());
}
}
Step 3: Automate browser testing with Selenium
Configure Selenium WebDriver to automate browser interactions, for example, automating the login process and verifying the user dashboard.
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class LoginIntegrationTest {
private WebDriver driver;
@Before
public void setUp() {
// Set path to ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
driver = new ChromeDriver();
}
@Test
public void testLogin() {
driver.get("http://localhost:8080/login");
// Enter username and password
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("password");
driver.findElement(By.id("loginButton")).click();
// Verify user is redirected to dashboard
String currentUrl = driver.getCurrentUrl();
assertEquals("http://localhost:8080/dashboard", currentUrl);
}
@After
public void tearDown() {
driver.quit();
}
}
Step 4: Run integration tests in Jenkins
Set up a Jenkins job to run the integration tests using Maven.
<project>
<builders>
<hudson.tasks.Maven>
<targets>clean verify</targets>
</hudson.tasks.Maven>
</builders>
</project>
Run the Jenkins pipeline. It will build the project, execute the JUnit and Selenium integration tests, and deploy the application if all tests pass.
Key takeaways
Integration testing remains a cornerstone in modern software development, particularly now when applications are becoming more complex and interconnected. By carefully validating the interactions between different modules, integration testing ensures that systems operate smoothly, delivering high-quality, reliable software products.
Implementing best practices, such as early planning, test automation, and continuous integration, as well as leveraging robust tools like Jenkins, Selenium, and JUnit, can significantly enhance the effectiveness of integration testing. As technology continues to advance, thorough and efficient integration testing will be key to achieving seamless and robust software solutions.
Ready to improve the quality of your software and ensure your systems work flawlessly together? Contact us to learn more about our integration testing services and how they can benefit your project.